Learn Spring - HelloWorld
Posted
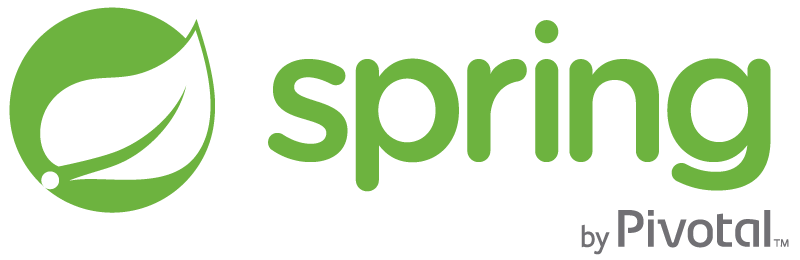
环境准备
- JDK 1.8+
- IntelliJ IDEA
- Spring框架(从Spring网站下载)
新建项目
使用下载的Spring框架新建项目
解压下载的框架,选择Use Library
,从解压的文件夹的libs
子文件夹选择需要的模块(也可以选择Download
等待IDEA
下载)
设置项目名
设置项目名为HelloWorld
。
添加代码
添加me.coolcodes
包
在src
文件夹下新建me.coolcodes
包。
添加HelloWorld.java
类
在me.coolcodes
包中添加HelloWorld
类
package me.coolcodes;
public class HelloWorld {
private String message;
public void setMessage(String message){
this.message = message;
}
public void getMessage(){
System.out.println("Your Message : " + message);
}
}
HelloWorld
类通过getMessage()
方法打印message
。
添加Main.java
类
在me.coolcodes
包中添加Main
类
package me.coolcodes;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml");
HelloWorld obj = (HelloWorld) context.getBean("helloWorld");
obj.getMessage();
}
}
Main
通过ClassPathXmlApplicationContext
读取Beans.xml
文件new
一个ApplicationContext
对象。context
通过ID创建一个Bean
(即HelloWorld对象),并转换成HelloWorld
对象。- 调用
HelloWorld
对象的getMessage()
方法打印message
。
添加Bean
文件(Beans.xml)
在src
文件夹下创建Beans.xml
配置文件
<?xml version = "1.0" encoding = "UTF-8"?>
<beans xmlns = "http://www.springframework.org/schema/beans"
xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation = "http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<bean id = "helloWorld" class = "me.coolcodes.HelloWorld">
<property name = "message" value = "Hello World!"/>
</bean>
</beans>
该xml
文件定义了一个Bean
,ID为helloWorld
,对应于me.coolcodes.HelloWorld
类。message
对应的值是Hello World!
。所以Main
中context
,通过getBean()
方法根据ID创建一个Bean
(即HelloWorld对象),并通过HelloWorld
的setMessage()
方法设置message
为Hello World!
。所以最后程序的输出应该是Hello World!
。
运行
运行配置
添加一个新的Application
配置,配置Main
类
结果
如前面所说,最后输出结果为Hello World!